Practical 2: BRDF models
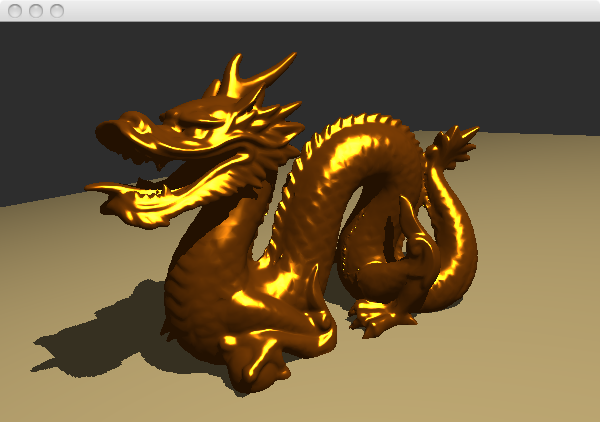
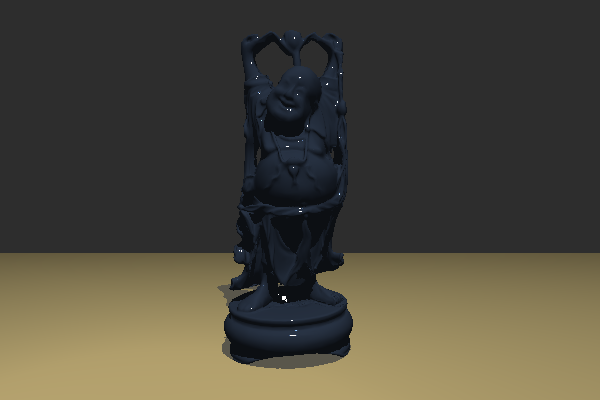
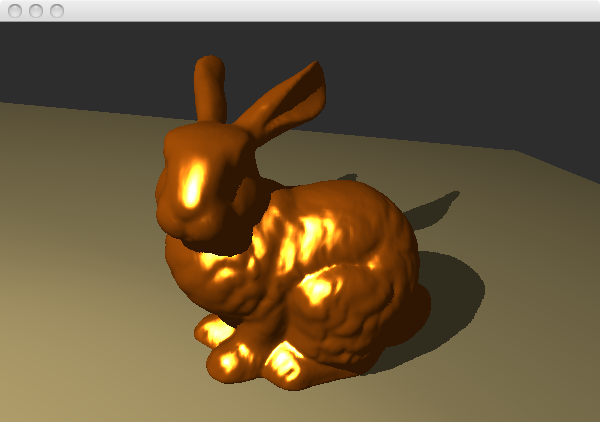
Summary of the Practical
The goal is to implement several BRDF models for per-pixel lighting using GLSL.We start with the result of the first practical. Make sure you have completed the first practical (and sent me your results!) before you start this one.
Instructions
We start with the shader you developed for the first practical. It displays objects with their shadows, computed using the shadow map method. We are going to extend it so that objects have a nice looking material. I suggest that you copy the shader you have done in a new directory (and, of course, edit Viewer.cpp so that it loads the new directory).
In order to compute a good BRDF (such as Blinn-Phong or Torrance), you will need the light vector (l=Light-Vertex), the view vector (v=Camera-Vertex) and the normal in the same coordinate system. Your current vertex shader computes the position of the light, the vertex and the normal in the coordinate system of the camera. The only thing missing is the position of the camera itself.
Note that the position of the camera in the coordinate system of the camera is not (0,0,0). You need to:
- create a new uniform variable (cameraPosition) in the vertex shader,
- have the program in Viewer.cpp set up the value of this uniform with the position of the camera in world space (camera()->position()),
- convert these coordinates in world space into coordinates in camera space.
Once you have done this, you have all the information required for the vertex shader to send: the light vector, the view vector and the normal to the fragment shader. It's better to normalize all three of them before leaving the vertex shader.
In the fragment shader, you'll have to normalize them again, since they have been interpolated. Especially, in order to compute the half vector, you'll need to normalize three times: once for the light vector and the view vector before you add them, and once for the result of the addition.
Having done all this, you now have all the information to run a Blinn-Phong shader. Try several values of the parameters (especially the power). The Experimental Analysis of BRDF Models Supplemental Document, at MIT, contains several parameter values corresponding to real materials.
When you're satisfied with the Blinn-Phong BRDF model, implement the Torrance BRDF. Use the formula in the Supplemental Document, with Schlick's approximation for Fresnel coefficients. Again, try several values of the parameters, corresponding to real materials. Compare the same material using both BRDF models (Blinn-Phong and Torrance). Can you spot the differences? the common points?
Once you're finished, send me an with: the source code for both shaders (vertex and fragment), as well as the screenshots.